Navagation基本用法
Navigation组件是路由导航的根视图容器,一般作为Page页面的根容器使用,其内部默认包含了标题栏,内容栏和公工具栏,其中内容区默认首页显示导航内容(Navigation的子组件)或非首页显示(NavDestination的子组件),首页和非首页通过路由进行切换
- 使用Navigation跳转的组件不需要再使用Entry来修饰,普通组件即可
- Navigation是一个导航组件,API9和API11的使用官方推荐方式各不相同
1. Navagation API9的用法-(Navigation-NavRouter-(其他组件+NavDestination的用法))
1. 1导航组件层次关系
组件 |
作用 |
层级规则 |
Navigation |
管理所有导航页面的根容器 |
必须包含多个 NavRouter |
NavRouter |
定义一个跳转入口 |
必须配对 NavDestination |
NavDestination |
目标页面内容 |
|
**1. **2 按钮覆盖问题
- 代码现象:
跳转B
覆盖 跳转A
- 根本原因:
在 NavRouter
内部放置多个直接同级组件时,仅最后一个组件会被渲染。
- ArkUI规则:
一个 NavRouter
只能关联 一个触发元素 (如Button),如需多个跳转需使用多个 NavRouter
。
1.3 代码案例
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35
| @Entry @Component struct TestNavgationAPI9 { build() {
Navigation() { NavRouter() { Button('跳转A') Button('跳转B') NavDestination() { Image($r('app.media.Coverpeople')) .width(50) .aspectRatio(1) NavRouter() { Text('我跳转啦') NavDestination() { Text('第三个界面') }
}
}
}
} .height('100%') .width('100%') } }
|
1.4 效果展示

2.Navagation 10的用法
2.1 路由栈自主管理
NavPathStack
核心方法:
1 2 3
| pushPath() replacePath() pop()
|
2.2 页面加载控制
navDestination(builder)
通过 Builder
函数动态渲染路由目标:
1
| .navDestination(this.navDesBuilder)
|
1 2 3 4 5 6
| @Builder navDesBuilder(name: string) { if (name === "pageTwo") { NavgationChild02() } }
|
2.3 跨组件通信
@Provide
与 @Consume
装饰器
1 2 3 4
| @Provide navPathStack: NavPathStack = new NavPathStack()
@Consume navPathStack: NavPathStack
|
无需显式传递,自动向下注入。
2.4 实现步骤
1. 自己管理页面栈 创建一个页面栈 (用@Provide修饰,方便跨组件使用)
1 2
| @Provide navPathStack: NavPathStack = new NavPathStack()
|
2.将创建的页面栈实例传递给主界面
ps
:这一步还是蛮关键的,很多页面不展示就是这个原因
1 2 3 4 5 6 7 8 9 10 11 12
| Navigation(this.navPathStack) { Text('这是第一页-首页') Button('去下一页') .onClick(() => { this.navPathStack.pushPath({ name: 'pageOne' }) })
} .height('100%') .width('100%')
|
3.通过 Builder
函数动态渲染路由目标
1. 先创建一个自定义Builder
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15
| @Builder myNavPathStackBuilder(name: string) { if (name === 'pageOne') { Children01()
} else if (name === 'pageTwo') { Children02()
} else if (name === 'pageThree') { Children03()
} }
|
2. 传入Builder
1
| .navDestination(this.navDesBuilder)
|
不需要加括号,这里有个底层原因
- ArkUI 的
navDestination
机制
- 设计逻辑:框架需要在导航时动态调用构建函数生成页面。若传递this.navDesBuilder()则:
- 代码执行时立即运行该函数(而非按需调用)
- 返回结果可能为
void
或非组件类型,导致渲染异常。
@Builder
函数的特性
3.跳转的实现
- 通过点击事件调用当前
navPathStack
实例pushPath
方法
- 传入你要跳转页面的名字,进行跳转
1 2 3 4 5 6
| Button('去下一页') .onClick(() => { this.navPathStack.pushPath({ name: 'pageOne' }) })
|
4.子界面的定义
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61
| @Component struct Children01 { @Consume navPathStack: NavPathStack build() { NavDestination() { Column({ space: 5 }) { Text('这是我从主页跳转的第一个界面') .fontSize(24) .fontWeight(500) Button('跳转到第二个页面去') .onClick(() => { this.navPathStack.pushPath({ name: 'pageTwo' }) }) } }
} } @Component struct Children02 { @Consume navPathStack: NavPathStack
build() { NavDestination() { Column({ space: 5 }) { Text('这是我从主页跳转的第二个界面') .fontSize(24) .fontWeight(500) Button('跳转到第三个页面去') .onClick(() => { this.navPathStack.pushPath({ name: 'pageThree' }) }) } }
} }
@Component struct Children03 { @Consume navPathStack: NavPathStack
build() { NavDestination() { Column() { Text('这是我从主页跳转的第三个界面') .fontSize(24) .fontWeight(500) }
}
} }
|
5.效果展示
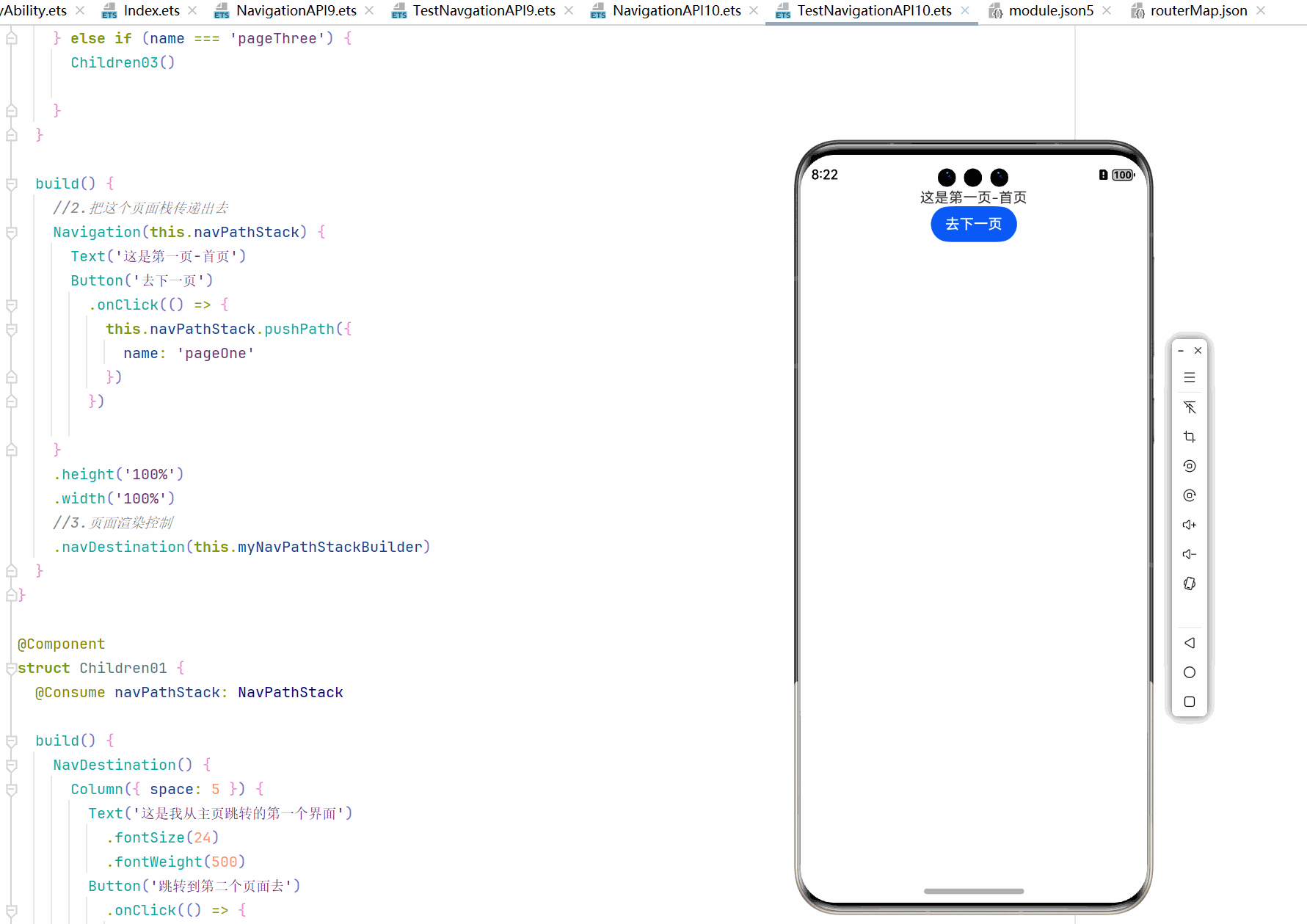
3.NavagationAPI10使用的补充内容
3.1 常见的API
配置组合 |
代码示例 |
效果描述 |
默认状态 |
Navigation() .title('主标题') |
标题栏显示 “主标题”,采用 Free 模式 |
精简模式+隐藏标题 |
Navigation() .titleMode(NavigationTitleMode.Mini) .hideTitleBar(true) |
标题栏完全不可见 |
子页面独立配置 |
NavDestination() .title('子页标题') .hideTitleBar(true) |
|
3.2 传参获取参数的实现
1.定义数据结构
1 2 3 4
| interface hobby { name: string, hobby: string }
|
2.即将跳转页面传入参数
1 2 3 4 5 6 7 8 9 10 11 12 13 14
| NavDestination() { Column({ space: 5 }) { Text('这是我从主页跳转的第二个界面') .fontSize(24) .fontWeight(500) Button('跳转到第三个页面去') .onClick(() => { this.navPathStack.pushPath({ name: 'pageThree', param: { name: '猫猫球', hobby: '喜欢玩毛线球' } as hobby }) }) } }
|
3.跳转的页面接收数据
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28
| @Component struct Children03 { @State hobby: hobby[] = [] as hobby[] @Consume navPathStack: NavPathStack
aboutToAppear(): void { this.hobby = this.navPathStack.getParamByName('pageThree') as hobby[] promptAction.showToast({ message: JSON.stringify(this.hobby) }) }
build() { NavDestination() { Column() { Text('这是我从主页跳转的第三个界面') Text('这是我获取的数据') Text(this.hobby[0].name) Text(this.hobby[0].hobby) .fontWeight(500) }
}
} }
|
4.效果展示
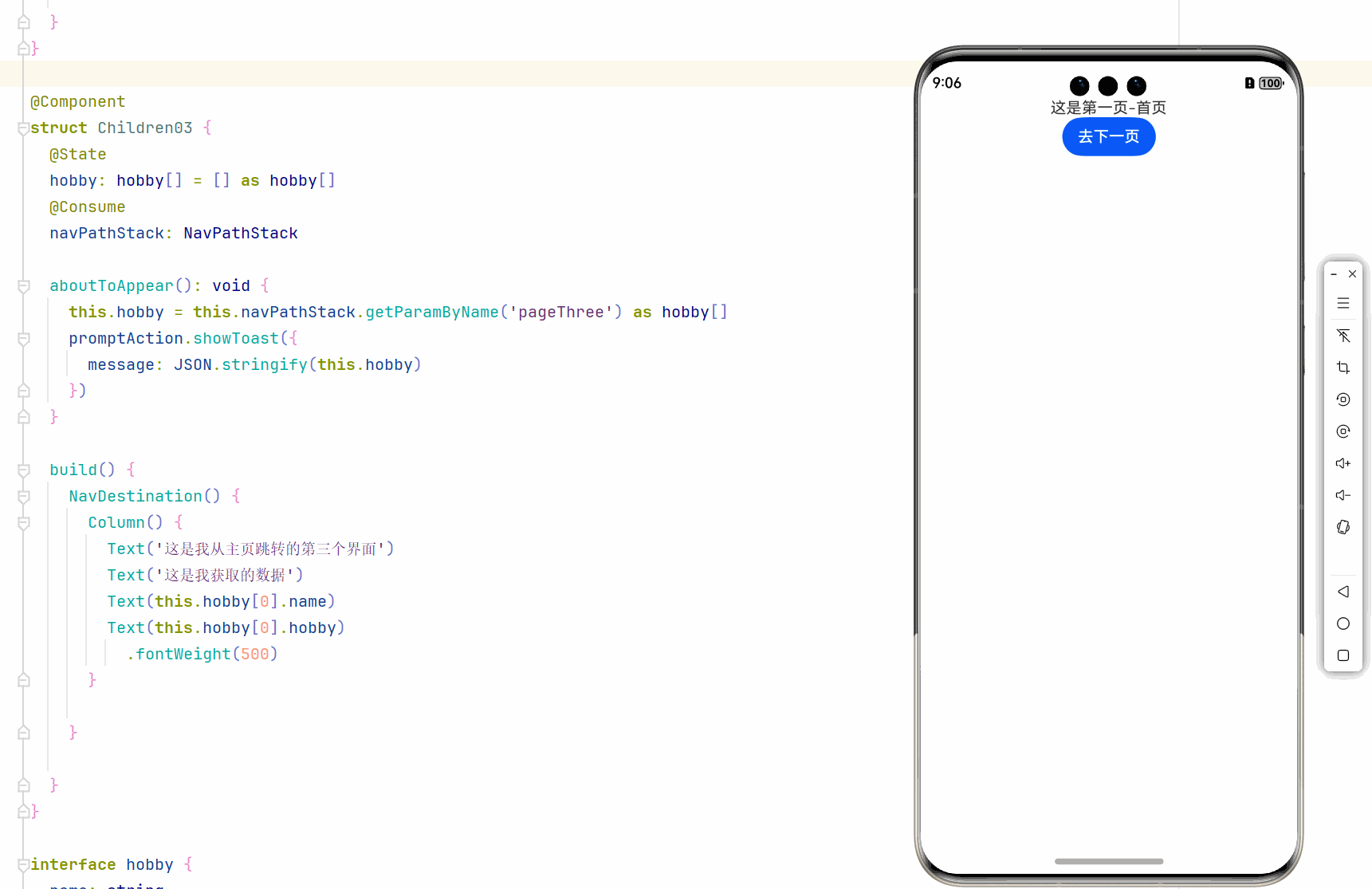